Hello! I’m Josep Gomis, and today I’m excited to share a simple Dice Simulator I created using Python. This project is a great way to practice Python while simulating the rolling of dice. It’s built in IDLE as I continue my Python learning journey. The code allows users to choose between rolling one or two dice, making it an interactive and fun tool.
Objectives
The goal of this project was to create a basic program that simulates the rolling of dice. The user can choose to roll one die or two dice. I used Python’s random module to simulate the randomness of dice rolls. It’s a great starting point for anyone learning Python.
Technologies Used
- Python: I used Python because it’s beginner-friendly and perfect for simple projects like this one.
- random module: The random module in Python generates random numbers, which is crucial for simulating dice rolls.
Code
Here’s the code I wrote for the Dice Simulator:
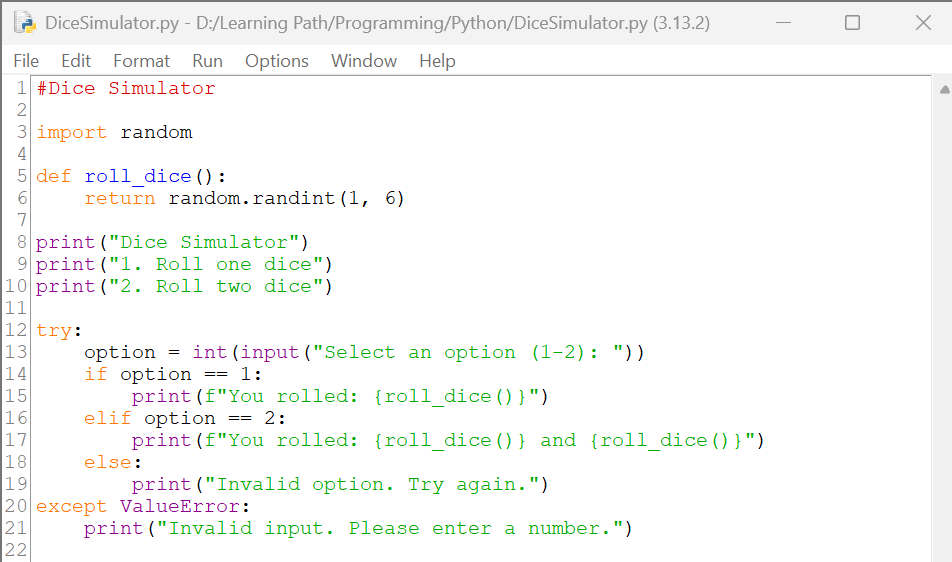
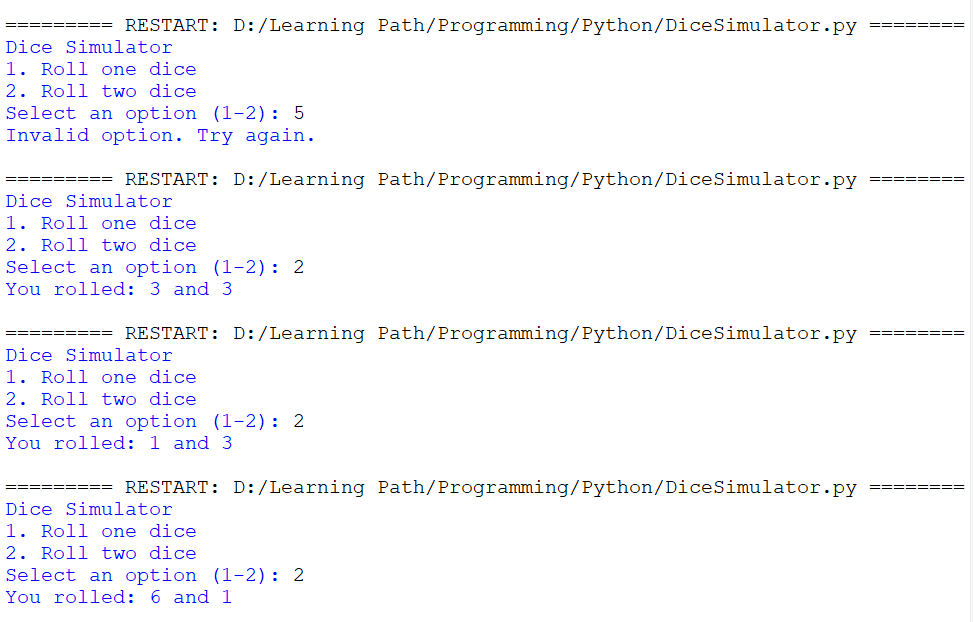
Explanation of the Code
import random
: This imports the random module, which is essential for generating random numbers.def roll_dice()
: This function simulates the rolling of a single 6-sided die by generating a random number between 1 and 6.- User Interaction: The program gives the user two options: roll one die or two dice. The user’s input determines the outcome, with the program printing the result of each roll.
- Error Handling: The
try-except
block ensures that the program handles invalid inputs gracefully by prompting the user to enter a valid option.
Possible Improvements
This project is straightforward but can be expanded:
- Multiple Dice Types: You could modify the program to support dice with more than 6 sides, like D20 or D4.
- Statistics: Track the number of rolls and calculate statistics such as the most frequent results.
- Graphical Interface: Adding a GUI using Python’s
tkinter
could make the simulator more interactive and visually appealing.
Conclusion
This Dice Simulator project was a great opportunity for me to practice Python. It’s a simple, fun project that demonstrates the basics of random number generation and user interaction. I look forward to building more Python projects in the future. Stay tuned for more!